Be the first user to complete this post
|
Add to List |
VBA-Excel: Change Font, Color, Weight of Table Data in the Word document
To Change Font, Color, Weight of Table Data in the Word document using Microsoft Excel, you need to follow the steps below (First we will create a table and while filling the data we change the font, color and weight of data):
- Create the object of Microsoft Word
- Create a document object and add documents to it
- Make the MS Word visible
- Create a Range object.
- Create Table using Range object and define no of rows and columns.
- Get the Table object
- Enable the borders of table using table object.
- Fill the data in table (Here we will insert some code to make changes in data)
- Save the document
Create the object of Microsoft Word
Set objWord = CreateObject(“Word.Application”)
Create a document object and add documents to it
Set objDoc = objWord.Documents.Add
Make the MS Word Visible
objWord.Visible = True
Create a Range object.
Set objRange = objDoc.Range
Create Table using Range object and define no of rows and columns.
objDoc.Tables.Add objRange, intNoOfRows, intNoOfColumns
Get the Table object
Set objTable = objDoc.Tables(1)
Enable the borders of table using table object.
objTable.Borders.Enable = True
Fill the data in table
objTable.Cell(1, 1).Range.Text = "Colored Data”
objTable.Cell(1, 1).Range.Font.Color = RGB(255, 0, 0)
objTable.Cell(1, 1).Range.Bold = True
Save the Document
objDoc.SaveAs ("D:\MyFirstSave")
Complete Code:
Function FnAddTableToWordDocument() Dim intNoOfRows Dim intNoOfColumns Dim objWord Dim objDoc Dim objRange Dim objTable intNoOfRows = 5 intNoOfColumns = 3 Set objWord = CreateObject("Word.Application") objWord.Visible = True Set objDoc = objWord.Documents.Add Set objRange = objDoc.Range objDoc.Tables.Add objRange, intNoOfRows, intNoOfColumns Set objTable = objDoc.Tables(1) objTable.Borders.Enable = True For i = 1 To intNoOfRows For j = 1 To intNoOfColumns objTable.Cell(i, j).Range.Text = "Colored Data_" & i & j objTable.Cell(i, j).Range.Font.Color = RGB(255, 0, 0) objTable.Cell(i, j).Range.Bold = True Next Next End Function
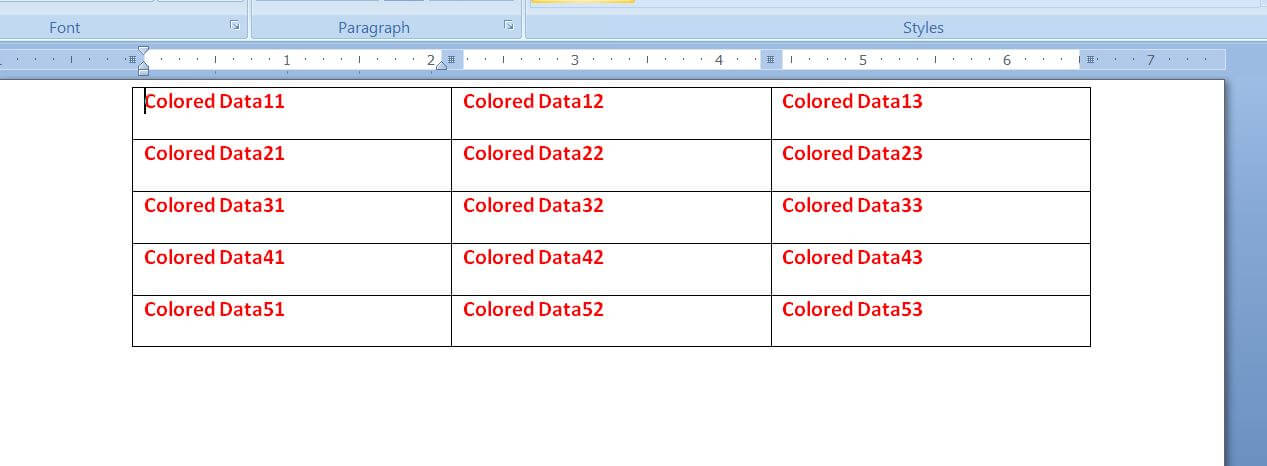
Also Read:
- VBA-Excel: Format already written text in a word document – Format All Content
- VBA-Excel: Create worksheets with Names in Specific Format/Pattern.
- Excel-VBA : Open a MS Word Document using Excel File using Explorer Window.
- VBA-Excel: Writing Text to Word document
- VBA-Excel: Writing Text to Word document