Be the first user to complete this post
|
Add to List |
VBA-Excel: Add Table and fill data to the Word document
VBA-Excel: Add Table and fill data to the Word document
To Add Table and fill data to the Word document using Microsoft Excel, you need to follow the steps below:
- Create the object of Microsoft Word
- Create a document object and add documents to it
- Make the MS Word visible
- Create a Range object.
- Create Table using Range object and define no of rows and columns.
- Get the Table object
- Enable the borders of table using table object.
- Fill the data in table
- Save the document
Create the object of Microsoft Word
Set objWord = CreateObject(“Word.Application”)
Create a document object and add documents to it
Set objDoc = objWord.Documents.Add
Make the MS Word Visible
objWord.Visible = True
Create a Range object.
Set objRange = objDoc.Range
Create Table using Range object and define no of rows and columns.
objDoc.Tables.Add objRange, intNoOfRows, intNoOfColumns
Get the Table object
Set objTable = objDoc.Tables(1)
Enable the borders of table using table object.
objTable.Borders.Enable = True
Fill the data in table
objTable.Cell(1, 1).Range.Text = "Sumit”
Save the Document
objDoc.SaveAs ("D:\MyFirstSave")
Complete Code:
Function FnAddTableToWordDocument() Dim intNoOfRows Dim intNoOfColumns Dim objWord Dim objDoc Dim objRange Dim objTable intNoOfRows = 5 intNoOfColumns = 3 Set objWord = CreateObject("Word.Application") objWord.Visible = True Set objDoc = objWord.Documents.Add Set objRange = objDoc.Range objDoc.Tables.Add objRange, intNoOfRows, intNoOfColumns Set objTable = objDoc.Tables(1) objTable.Borders.Enable = True For i = 1 To intNoOfRows For j = 1 To intNoOfColumns objTable.Cell(i, j).Range.Text = "Sumit_" & i & j Next Next End Function
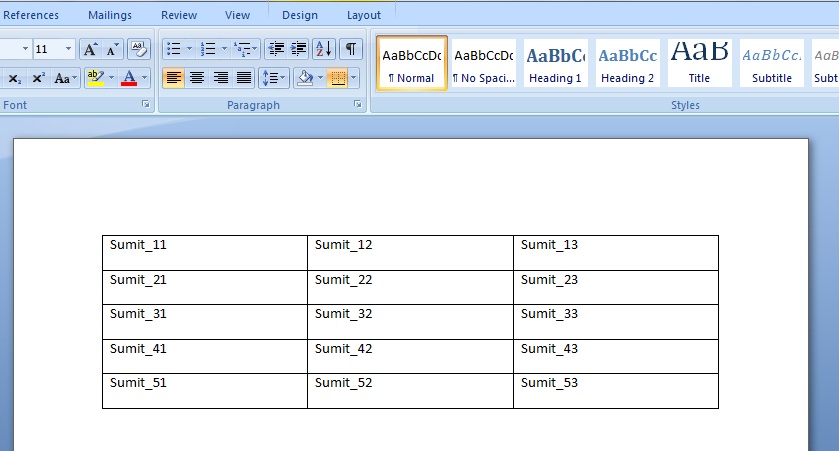
Also Read:
- VBA-Excel: Add Worksheets For All The Given Dates Except Weekends and Copy The Common Template In Each Worksheet
- VBA-Excel: Create worksheets with Names in Specific Format/Pattern.
- VBA-Excel: Get ALL The Opened Internet Explorer (IE) using Microsoft Excel
- VBA-Excel: Edit And Save an Existing Word Document
- Excel-VBA : Send a Mail using Predefined Template From MS Outlook Using Excel